- Go to https://colab.research.google.com/
Create a new notebook (I have mine saved in Drive) - Create a code cell and run the following code:
from google.colab import drive
drive.mount('/content/drive')
You will be asked to give Colab permission to access your Drive. If you have previously given permission, you still need to click through the entire prompt.
If this is successful, you will see your drive mounted in /content/drive in the file explorer on the left side of the notebook:
You should now be able to browse your files.
NOTE: If you want to share something from ‘Shared with Me’ or something that is not in the root level of your drive, the easiest way to access this is to make a shortcut and put it in your root level drive directory:
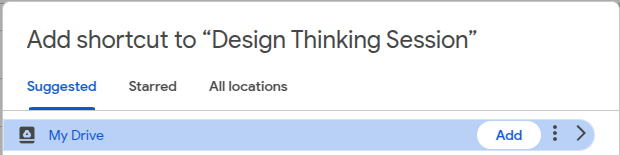
It will then show up in the file explorer to the left of the notebook:
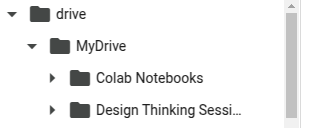
It looks like this in your actual Drive UI:
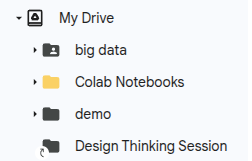

You can then interact with the files with normal OS python code, such as:
import os
directory_path = '/content/drive/MyDrive/some/folder'
def list_files_recursively(f, path):
for root, dirs, files in os.walk(path):
for file in files:
f.write(os.path.join(root, file))
with open('/content/drive/MyDrive/file-listing.txt', 'w') as f:
list_files_recursively(f, directory_path)